Getting User Consent Before Profiling
Making it easier to meet the Google Play Program Policies
Before your application transmits data back to PushSpring, it should inform your users and get consent. For more info see Google's Developer Policy Center: https://play.google.com/about/privacy-security/user-data/. To help bootstrap this process, the PushSpring SDK contains a convenience method to facilitate this process. Simply call:
PushSpring.showServiceAgreementDialog(
@NonNull Activity activity,
@Nullable ServiceAgreementAcceptanceListener listener,
@Nullable URL privacyPolicyUrl,
@Nullable String messageOverride,
@Nullable URL termsOfServiceUrl);
to display a Dialog similar to the one below:
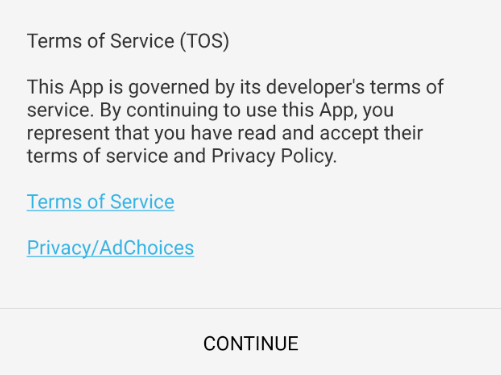
Optional arguments
Notice that not all arguments are required. The @Nullable arguments are intended to help developers tweak the Dialog, not fully configure it. If more control is required, it's recommended that developers create their own dialog or other UI to display the enhanced notice regarding data collection.
An optional ServiceAgreementAcceptanceListener can be used monitor the onAcceptanceClick() and persist the state of user consent. Here is one example:
@Override
public void onAcceptanceClick() {
final SharedPreferences.Editor editor = getApplicationContext()
.getSharedPreferences("YourPrefs", Context.MODE_PRIVATE)
.edit();
editor.putBoolean("userConsents", true);
editor.apply();
}
This flag can be checked in your Activity's onCreate() method to prevent data transmission until the user has acknowledged it.
public class MainActivity extends Activity implements ServiceAgreementAcceptanceListener {
...
@Override
protected void onCreate(Bundle savedInstanceState) {
final SharedPreferences preferences = getApplicationContext().getSharedPreferences("YourPrefs", Context.MODE_PRIVATE);
boolean userAcceptedTOS = preferences.getBoolean("userConsents", false);
PushSpring.sharedPushSpring().setTransmissionAllowed(userAcceptedTOS);
if (!userAcceptedTOS) {
serviceAgreementDialog = PushSpring.showServiceAgreementDialog(this, this, null, null, null);
}
}
...
}
For a more in-depth example please see the PushSpringSDKSample project that accompanies the SDK.
Updated less than a minute ago